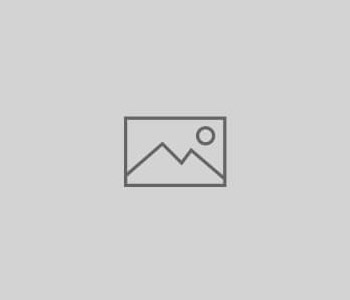
Tutorial 04 – Animated character: Idle, Move, Run!
In this tutorial I’ll show you how to easily change the GO Map character with an animated 3D model.
I’m going to use the “Hellephant” model from the Unity “Zombie Toys” game that’s available freely on the asset store.
Bye the way “Zombie Toys” is a very nice project to learn the unity basics and I suggest you to take a look at it.
There’s also a courseware that’s entirely about it’s making and it’s really great.
Why the Hellephant and not the “Zombie Toys” player character?
I mean have you seen it? It’s just so cool.
Set up the character
Let’s start by importing the Hellephant model into the project and set up the animator controller. You can import the model wither from the zombie toys project or from this unitypackage.
If you use the package above you’ll find everything you need in it, otherwise you’ll maybe need to cut the animations and create an animator controller that looks like this.
*Remember to make the idle and the move animation without exit time and looping*
Set up the scene
Now that you have set up the model let’s import it into the scene as a prefab. However there’s already a scene with everything set up in the unitypackage above.
Put the hellephant prefab at the same level of the GoMap Character in the hierarchy. And Check that it’s inspector looks like this, with a “Animate Character” component attached to it.
Set up the Avatar: OnAnimationStateChanged Event
Now select the Avatar gameobject in the hierarchy and drag the Hellephant prefab in the “Avatar Figure” property.
In the OnAnimationStateChanged Event Panel at the bottom of the inspector you just have to click on the plus icon to add a new listener, drag the Hellephant gameobject in the object property and select the “OnAnimationStateChange” method of the AnimateCharacter component. That will do the trick, sending animation state change events to our simple script.
AnimateCharacter.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | using System.Collections; using System.Collections.Generic; using UnityEngine; using GoShared; public class AnimateCharacter : MonoBehaviour { Animator animator; void Start () { animator = GetComponent(); } public void OnAnimationStateChange (MoveAvatar.AvatarAnimationState animationState) { switch (animationState) { case MoveAvatar.AvatarAnimationState.Walk : case MoveAvatar.AvatarAnimationState.Run : animator.SetBool("isMoving", true); break; case MoveAvatar.AvatarAnimationState.Idle : animator.SetBool("isMoving", false); break; } } } |
This script is quite simple. It just implements a method which has a switch for the different animation states and triggers the right animation.
Job done, that easy. The MoveAvatar script basically does all the work for you.
In this way when you move with GPS updates your character will animate accordingly.
The possible states are:
Idle
Walk
Run
Based on your real world speed. Sadly the hellephant only has a move animation without a run animation.
I’ll leave to you the setup of a model with 3 animations editing the AnimateCharacter script.
Bye
Alan